The Purpose of the Article
The purpose of this article is to provide a comprehensive guide on implementing CRUD (Create, Read, Update, Delete) operations using FastAPI, a modern, high-performance web framework for building APIs with Python. By the end of the article, readers will understand how to set up a FastAPI project, configure a database, define data models and schemas, and create API endpoints to handle CRUD operations. This guide aims to equip developers with practical knowledge and examples to build robust and scalable APIs efficiently with FastAPI. So let us begin now to learn FastAPI.
Introduction to FastAPI:
FastAPI is a modern, high-performance web framework for building APIs with Python
3.6+ based on standard Python type hints. It’s designed to be easy to use and offers the best possible developer experience, including efficient error handling and clear code structure. FastAPI simplifies API development, providing automatic documentation and high performance, making it a popular choice for building robust and scalable web applications.
Advantages of FastAPI:
- High Performance
- Ease of Use
- Automatic Data Validation
- Standards-Based
- Dependency Injection
- Asynchronous Support
- Developer Productivity
- Built-in Security
- Extensibility
- Active Community and Ecosystem.
Explanation of CRUD Operations (Create, Read, Update, Delete):
CRUD operations are the four basic functions of persistent storage, often associated with database management and RESTful APIs. They represent the foundational actions that can be performed on a data set.
- Create
Create operations add new records to a database or data storage system. This typically involves submitting data through an API endpoint, which then inserts the new data into the database.
Example:
HTTP Method: `POST` Endpoint: `/items/`
Function: Adds a new item to the database.
- Read
Read operations retrieve existing records from the database. This can be done for a single record or multiple records and is commonly used to display data to users or provide data for other systems.
Example:
HTTP Method: `GET`
Endpoint: `/items/` (for all items) or `/items/{id}` (for a specific item) Function: Fetches and returns the requested data.
- Update
Update operations modify existing records in the database. This involves sending updated data through an API endpoint, which then updates the corresponding record in the database.
Example:
HTTP Method: `PUT` or `PATCH` Endpoint: `/items/{id}`
Function: Updates an existing item with new data.
- Delete
Delete operations remove existing records from the database. This is typically done through an API endpoint, which deletes the specified record from the database.
Example:
HTTP Method: `DELETE` Endpoint: `/items/{id}`
Function: Deletes the specified item from the database.
Understanding these CRUD operations is fundamental to working with databases and APIs, as they form the backbone of data manipulation and management in most applications.
Structuring of FastAPI based on File-Type:
In this approach, files are organized by type (e.g., API, CRUD, models, schemas, routers) as represented by FastAPI iteslf.
This structure is more suitable for microservices or projects with fewer scopes:
my_fastapi_project/
├── app/
│ ├── init .py
│ ├── main.py
│ ├── dependencies.py
│ ├── routers/
│ │ ├── init .py
│ │ ├── items.py
│ ├── crud/
│ │ ├── init .py
│ │ ├── items.py
│ ├── schemas/
│ │ ├── init .py
│ │ ├── item.py
│ ├── models/
│ │ ├── init .py
│ │ ├── item.py
│ ├── external_services/
│ │ ├── init .py
│ ├── utils/
│ │ ├── init .py
├── tests/
│ ├── init .py
│ ├── test_items.py
├── database.py
├── requirements.txt
├── .env
└── README.md
In this structure:
app/: Contains the main application files. main.py: Initializes the FastAPI app.
dependencies.py: Defines dependencies used by the routers. routers/: Contains router modules.
crud/: Contains CRUD (Create, Read, Update, Delete) operation modules. schemas/: Contains Pydantic schema modules.
models/: Contains database model modules.
external_services/: Contains modules for interacting with external services.
Utils/: contains utility modules.
Test/: contains test modules.
│ │ ├── init .py
│ │ ├── items.py
│ ├── schemas/
│ │ ├── init .py
│ │ ├── item.py
│ ├── models/
│ │ ├── init .py
│ │ ├── item.py
│ ├── external_services/
│ │ ├── init .py
│ ├── utils/
│ │ ├── init .py
├── tests/
│ ├── init .py
│ ├── test_items.py
├── database.py
├── requirements.txt
├── .env
└── README.md
In this structure:
app/: Contains the main application files. main.py: Initializes the FastAPI app.
dependencies.py: Defines dependencies used by the routers. routers/: Contains router modules.
crud/: Contains CRUD (Create, Read, Update, Delete) operation modules. schemas/: Contains Pydantic schema modules.
models/: Contains database model modules.
external_services/: Contains modules for interacting with external services.
Utils/: contains utility modules.
Test/: contains test modules.
Connecting with PostgreSQL in FastAPI:
Connecting FastAPI to a PostgreSQL database involves setting up the database connection using SQLAlchemy and the `psycopg2` driver. These are the following steps on how to configure PostgreSQL with FastAPI:
- Install Required Packages
First, you need to install SQLAlchemy and `psycopg2`:

- Database Configuration
Create a `database.py` file to handle the database connection and session management.
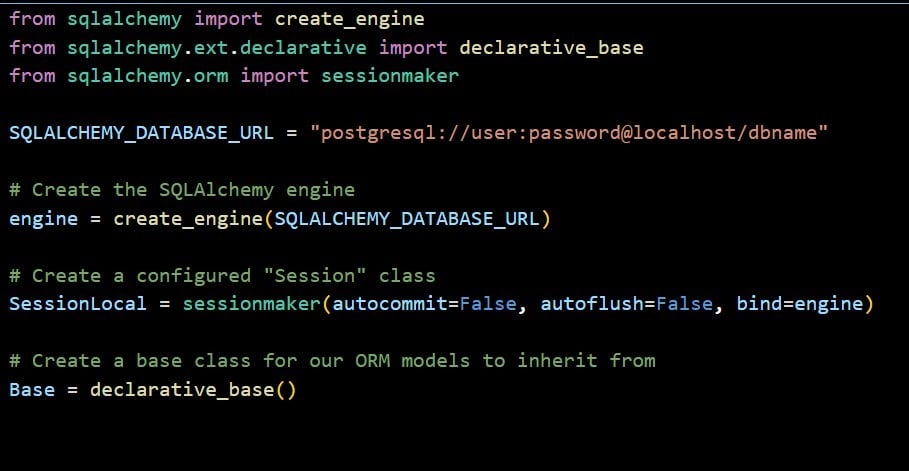
Connecting with PostgreSQL in FastAPI:
Connecting FastAPI to a PostgreSQL database involves setting up the database connection using SQLAlchemy and the `psycopg2` driver. These are the following steps on how to configure PostgreSQL with FastAPI:
- Install Required Packages
First, you need to install SQLAlchemy and `psycopg2`:

- Database Configuration
Create a `database.py` file to handle the database connection and session management.
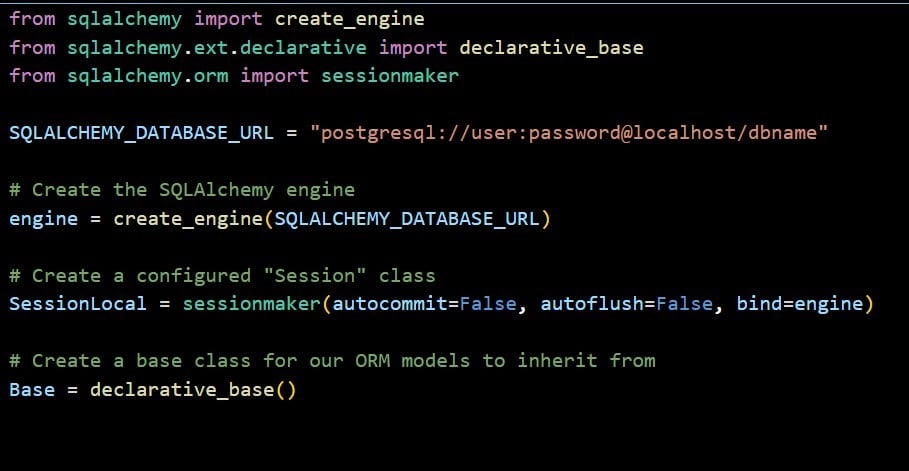