Overview of Testing: –
Testing is essential in software development because it helps us find and fix problems early. This article will teach you how to write unit tests and API test cases for FastAPI applications using Python. Whether you’re new to programming or just new to testing, this guide will provide you with the knowledge you need to get started.
Table of Contents:
- Abstract
- Introduction to Testing in FastAPI
- Setting Up the Environment
- Writing Testcases
- Advanced Features
- Advanced Topics
- Conclusion
- Abstract:- In this article, we will explore how to write unit tests and API test cases for FastAPI applications using Python. Testing is a crucial part of software development that ensures your code works correctly and is free from defects. By the end of this guide, you will have a good understanding of setting up your testing environment, writing various types of tests, and following best practices to maintain high code quality.
- Introduction to Testing in FastAPI:-
- Overview of FastAPI:
- FastAPI is a modern web framework first released in 2018 for building RESTful APIs in Python. It is used for building APIs with Python 3.8+ based on standard Python-type hints. FastAPI uses type hints to validate, serialize and deserialize data. Thus, it is based on Pydantic in that it allows custom data types to be defined or you can extend validation with methods, on a model decorated with the validator decorator.
- Features of FastAPI:
- Fast: As the name suggests, FastAPI is fast. It can handle a lot of requests quickly, making it suitable for high-performance applications.
- Easy to Use: FastAPI uses standard Python type hints, which helps us to write less code while still getting powerful features. This makes the code easier to read and maintain.
- Automatic Documentation: FastAPI automatically generates interactive documentation for our API, so you can easily see and test the API endpoints in our browser.
- Asynchronous Support: FastAPI supports asynchronous programming, which allows our application to handle many tasks at the same time, improving performance and responsiveness.
- Type Safety: With Python type hints, FastAPI provides validation and serialization out of the box, ensuring our data is always in the expected format.
- Type of Test Cases:-
- Unit Tests:
- These tests focus on individual functions, routes, or small pieces of our application logic.
- They are typically isolated and don’t interact with external dependencies like databases or external services.
- Unit tests help ensure the correctness of individual components of our application.
2) Functional Tests:
- These tests simulate user interactions with the API by sending HTTP requests and verifying the responses.
- They test the overall functionality of our API endpoints from an external perspective.
- Functional tests help ensure the API behaves as intended for real users.
3) Integration Tests:
- These tests verify how different parts of our application work together.
- They may involve testing interactions with databases, external APIs, or other services our application relies on.
- Integration tests provide more confidence that our application functions as expected.
4) Acceptance Tests:
- These are high-level tests that represent real-world user scenarios.
- They may involve user interface (UI) automation tools or other tools to simulate user interactions with the application.
- Acceptance tests provide broader validation of our application’s functionality from a user’s standpoint.
- Setting Up the Environment:-
- Installing Necessary Packages:-
- Make sure you have installed Python and FastAPI on your system. Here, we are installing a few other packages for testing, so the preferable way is to create a virtual environment and then install those packages inside it.
- Create venv – python3 –m venv venv_path/.venv (env name)
- Activate venv:- .venv\Script\activate.
- You can install the packages below using ‘pip install pkg_name‘.
- pytest: The main testing framework.
- httpx: A client for testing FastAPI endpoints.
- Uvicorn: An ASGI (Asynchronous Server Gateway Interface) server for running FastAPI.
- File Structure:- We are following the below file structure for our app.
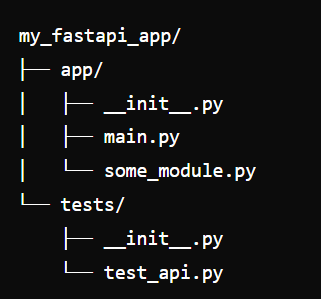
- Writing Test Cases:-
- In this article we are going to cover unit and functional testing.
1) Unit Testing:-
- It involves testing individual components of our application, such as functions or methods, to ensure they work correctly in isolation. By writing unit tests, we can catch bugs early, simplify debugging, and maintain high code quality. Using tools like ‘pytest’, we can write and run these tests efficiently, ensuring our FastAPI application remains reliable and robust.
- Pytest:- pytest is a popular testing framework for Python. It makes it easy to write simple and scalable test cases, helping you ensure your code works correctly. You can by just writing ‘./python file_name.py’ command for specific file and run ‘pytest’ against the whole dir of tests.
- Key characteristics of Unit Tests:
- Isolation: Unit tests isolate the unit under test from the rest of the application. This allows for focused testing without worrying about external factors.
- Dependency Injection: FastAPI’s dependency injection system is a powerful tool for unit testing. You can leverage it to mock or override dependencies (like database connections) during testing, ensuring your unit test solely focuses on the functionality being tested.
- Focus on Logic: Unit tests primarily target the internal logic of your application, verifying its behavior with specific inputs and expected outputs.
- Benefits of Unit Tests:
- Early Error Detection: Unit tests can help identify issues early in the development process, leading to faster bug fixing and a more robust codebase.
- Improved Code Maintainability: Well-written unit tests act as documentation, clarifying the purpose and behavior of individual functionalities. This makes code maintenance and future modifications easier.
- Increased Confidence: Unit tests provide a safety net by ensuring core functionalities work as intended. This builds confidence in your application’s overall behavior.
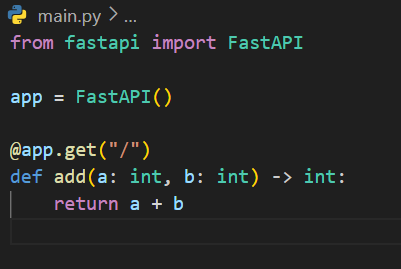
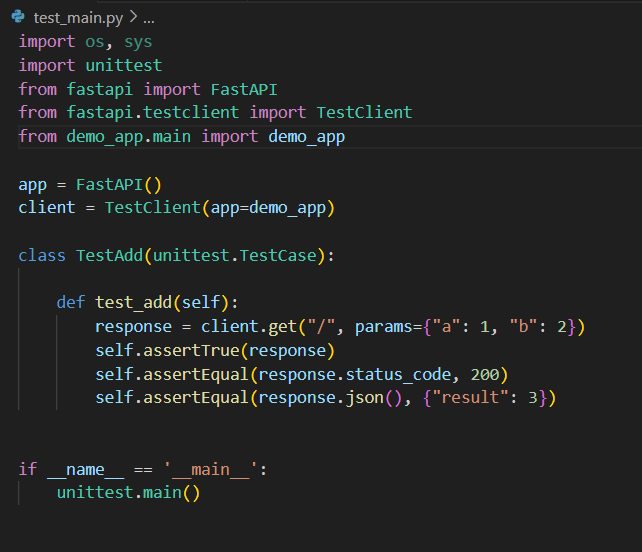
- Async Testing:- Async tests are designed to test asynchronous code, which is common in FastAPI applications. Using IsolatedAsyncioTestCase, we can write tests that handle async functions, ensuring our application’s asynchronous operations work correctly. This helps maintain the performance and reliability of your FastAPI endpoints which rely on async processing.
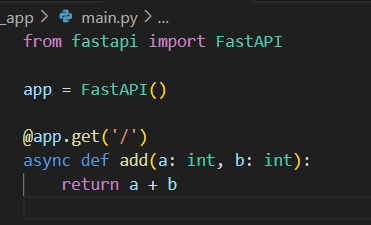
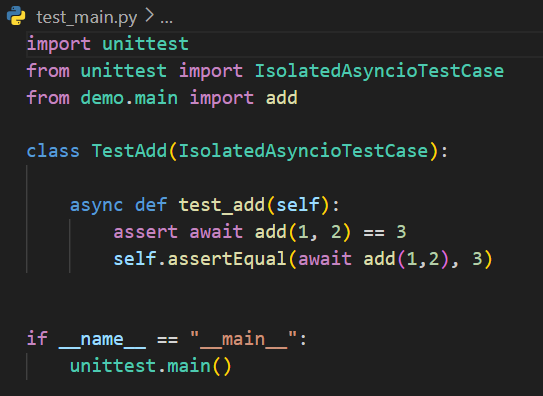
- Functional Tests:-
- Functional tests simulate real user interactions with our FastAPI application by sending HTTP requests and verifying the responses. They essentially test the API’s functionality from an external perspective, mimicking how a user would interact with it.
- Key characteristics of Functional Tests:
- External Perspective: Unlike unit tests that isolate functionalities, functional tests operate from outside the application, treating the API as a black box.
- HTTP Request-Response Validation: They focus on sending various HTTP requests (GET, POST, PUT, etc.) to the API endpoints and ensuring the received responses match expectations (correct status code, data structure, etc.).
- User-Centric Approach: By simulating user interactions, functional tests provide confidence that the API behaves as intended for real users.
- Benefits of Functional Tests:
- Higher-level Validation: Compared to unit tests that focus on individual components, functional tests validate the overall functionality of API endpoints.
- Real-world Simulation: They mimic user interactions, offering valuable insights into how the API behaves in practical scenarios.
- Improved API Quality: By catching issues that might affect user experience, functional tests contribute to a more robust and reliable API.
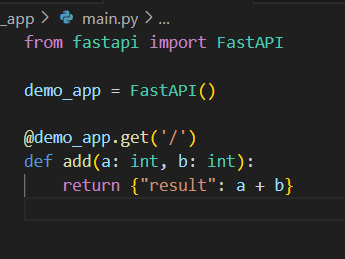
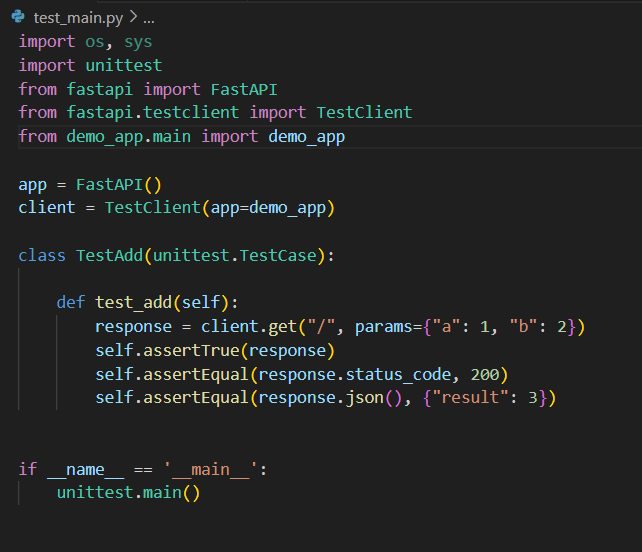
- Advanced Features: Power Up the FastAPI App
- FastAPI goes beyond the basics! This section dives into some of the more advanced features that can take your application to the next level. Here are some examples:
- Dependency Injection: Imagine having ingredients prepped and ready for your recipes. Dependency injection works similarly, providing pre-configured objects and data that your routes and functions need to operate. This makes your code cleaner and easier to test.
- Background Tasks: Need to send an email notification after a user signs up? FastAPI lets you handle tasks like this in the background, keeping your API responsive for users.
- Automatic Documentation: Wouldn’t it be great if your API automatically generated its own user guide? FastAPI boasts built-in features that create interactive docs based on your code, saving you time and effort.
- These are just a few examples. Advanced features in FastAPI offer a toolbox for building more complex and powerful applications.
- Advanced Topics: Deepen Your FastAPI Knowledge
- Ready to explore even more? FastAPI has a rich ecosystem with advanced topics that cater to specific needs. Here are a couple to consider:
- Security: Protecting your API from unauthorized access is crucial. FastAPI offers tools and techniques to implement robust security measures like authentication and authorization.
- Custom Middlewares: Imagine having a special checkpoint for all incoming requests. Middleware in FastAPI allows you to intercept requests and responses, adding custom logic to fine-tune your application’s behavior.
- These advanced areas empower you to tackle intricate challenges and build secure, feature-rich APIs.
- Conclusion:
- In this article, we explored the essential aspects of testing in FastAPI. We began with an introduction to the importance and basics of testing. Setting up the environment was the next step, ensuring a smooth start for writing tests. We delved into creating unit tests and functional tests, showcasing how to validate individual components and overall application behavior. Additionally, we touched on some advanced features and topics to enhance testing practices further.