Industry is trending toward splitting your monolithic application into microservices to segregate data, code, and interfaces. It’s not always an easy task to accomplish, especially if you don’t have any experience in Microservice development, best practices, and essential design patterns and principles.
Let’s take a deep dive and learn how this could be done with some excellent design patterns.
Service Registry:
The Service Registry Pattern provides a central repository to discover Microservices by name. It is a microservice architecture pattern that enables services to discover other Microservices and communicate with each other.
In this pattern, a central service registry or directory is used to keep a record of the available services and their locations. Microservices can register themselves with the registry, and other Microservices can look up the registry to find the location of services they may require.
For example, suppose we have a large e-commerce website that consists of many microservices, such as an order service, a payment service, a shipping service, and a customer service. Each of these services has its own REST API that other services can use to communicate with it.
- To make it easier for these services to discover each other, we can use the Service Registry Pattern. We can set up a service registry, such as Eureka Server, that maintains a list of all the available services and their endpoints.
- When a service starts up, it can register itself with the registry by providing its name and endpoint.
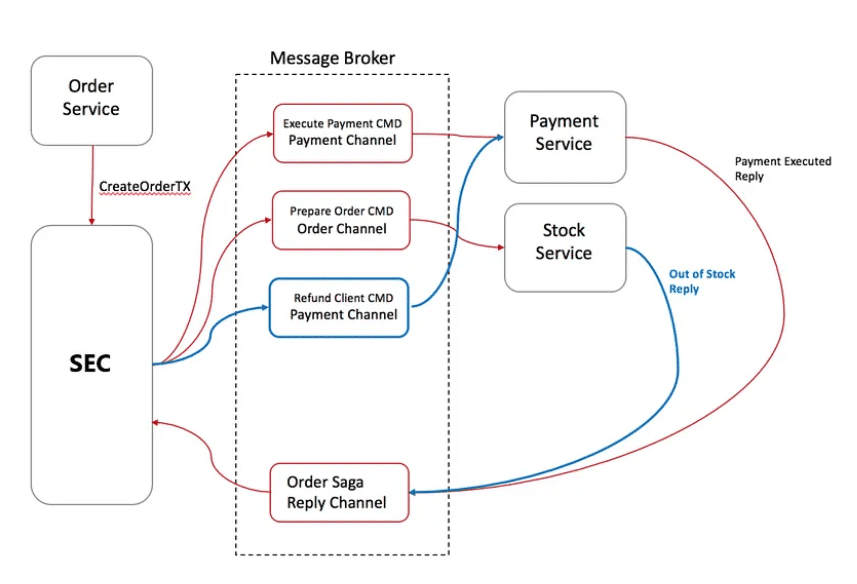
Circuit Breaker Pattern:
As the name suggests, the Circuit Breaker pattern prevents cascading failures by breaking a circuit (i.e. like a circuit breaker in an electrical box that stops the flow of electricity when the circuit can’t handle the load). This pattern enables applications to continue functioning when one or more services fail. It is used to handle faults that may occur in a microservice architecture.
In this pattern, a circuit breaker acts as a safety net between the client and the service, protecting the client from excessive and cascading failures in your service.
The circuit breaker monitors the status of the outbound call health and, if it detects that the service is failing, it will open the circuit and prevent further requests from being sent to the service until the service has recovered.
It can also send some traffic when the state of the circuit is half-open allowing it to determine if the underlying dependency is still down or if additional traffic can now be sent again.
For example, suppose a microservice application is using an external service that is unreliable, and the application needs to continue functioning even if the external service is failing.
- In this scenario, the Circuit Breaker pattern can be used to detect when the external service is unavailable and switch to an alternative service or a fallback service until the external service becomes available again.
- In a Microservice architecture, the Circuit Breaker pattern can be implemented using tools such as Netflix’s Hystrix, Resilience4j, or Spring Cloud Circuit Breaker. Each of these implementations provide a way to manage the circuit breaker’s behaviour and allow the application to react to service failures in a controlled manner.
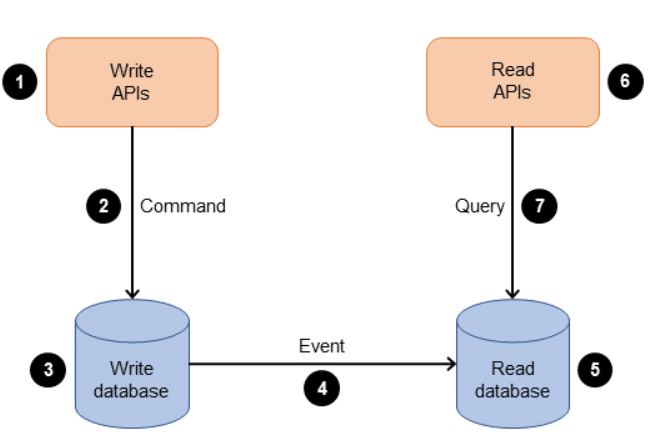
API Gateway Pattern:
The API Gateway Pattern is another common design pattern used in microservices architecture that involves an API gateway. The API Gateway acts as a single point of entry for all incoming API requests. The API Gateway then acts as a proxy between the clients and the microservices, routing requests to the appropriate service and endpoint.
The main purpose of an API gateway is to decouple the clients from the microservices, abstracting the complexity of the system behind a simplified and consistent API. This also means that you don’t need to find and remember the URLs of a 100+ Microservice REST APIs.
It also provides an additional layer of security and governance, allowing organizations to control and manage access to their services, monitor the performance of the system, and enforce policies across all the services.
Here is an example of how the API Gateway pattern works in a simple e-commerce system:
- Suppose an e-commerce system has multiple microservices to handle different functions such as order management, product catalogue, and user authentication. Each microservice has its own API endpoint for handling requests. However, the client, which could be a web or mobile application, needs to access all these microservices through a single-entry point.
- This is where an API Gateway comes into play. The API Gateway acts as a reverse proxy that receives all incoming requests from the clients. It then routes each request to the appropriate microservice based on the endpoint requested.
- For example, an API Gateway might route requests to an /orders endpoint in the order management microservice, and then makes requests to /products endpoint in the product catalogue microservice.
The API Gateway can also perform additional functionality, such as request and response transformation, rate limiting, authentication and authorization, as well as caching.
Overall, the API Gateway pattern provides a scalable, flexible, and secure way to manage microservices in a complex system, making it easier to develop, deploy, and maintain Microservices-based applications.
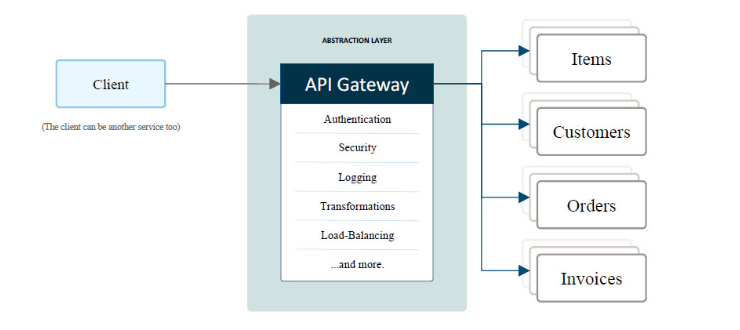
Saga Pattern:
The Saga Pattern provides a way to manage transactions that involve multiple microservices. It is used to ensure that a series of transactions across multiple services are completed successfully, and if not, to roll back or undo all changes that have been made up to that point.
The Saga pattern consists of a sequence of local transactions, each of which updates the state of a single service, and a corresponding set of compensating transactions that are used to undo the effects of the original transactions in case of a failure.
For example, suppose you have two microservices: one responsible for processing orders and another responsible for shipping orders:
- When a new order is placed, the order processing service is responsible for validating the order and ensuring that the items are in stock, while the shipping service is responsible for packaging the order and sending it to the customer.
- If the order processing service determines that the order is valid and all items are in stock, it sends a message to the shipping service to initiate the shipping process. At this point, the Saga pattern comes into play.
- The shipping service will create a new transaction to package and ship the order, and if the transaction is successful, it will mark the order as shipped.
- If, on the other hand, the transaction fails (perhaps due to a problem with the shipping provider), the shipping service will initiate a compensating transaction to undo the effects of the original transaction, such as cancelling the shipment and restocking the items.
- Meanwhile, the order processing service is also using the Saga pattern to manage its own transaction. If the shipping service reports that the order has been shipped successfully, the order processing service will mark the order as completed.
- If the shipping service reports a failure, the order processing service will initiate a compensating transaction to cancel the order and return any funds that were paid.
Overall, the Saga pattern provides a way to manage complex transactions across multiple microservices in a way that ensures consistency and reliability. If can only learn one pattern, might I suggest the SAGA patterns as its immensely helpful in Microservice applications.
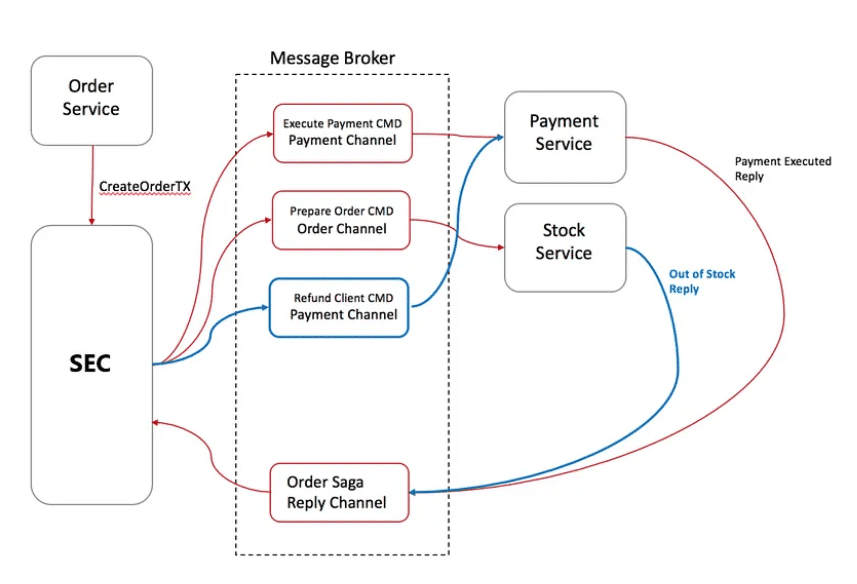
Command Query Responsibility Segregation (CQRS) Pattern:
CQRS is another popular Microservice design pattern that separates commands (write operations) and queries (read operations) into separate models, each with its own database.
In this pattern, a Command Model receives commands from the client and writes to the database. The Query Model reads from the database and sends data to the client. The pattern can be used to improve the performance and scalability of a system, as each model can be optimized for its specific task.
For example, consider an e-commerce application that uses a traditional CRUD-based approach to managing product information. The same model and database are used to handle both reading and writing product information. As the application grows, the model becomes increasingly complex and the database becomes a performance bottleneck.
- Using CQRS, the application would have a separate Command Model for writing product information, and a separate Query Model for reading product information. The Command Model would be optimized for fast writes, while the Query Model would be optimized for fast reads.
- The Command Model would store data in a write-optimized database, while the Query Model would store data in a read-optimized database. The two models would communicate through an event bus or message queue.
Overall, CQRS can improve the scalability and performance of a system, as well as simplify the code base by separating concerns. However, it can also add complexity and require more development effort, as separate models and databases are required.
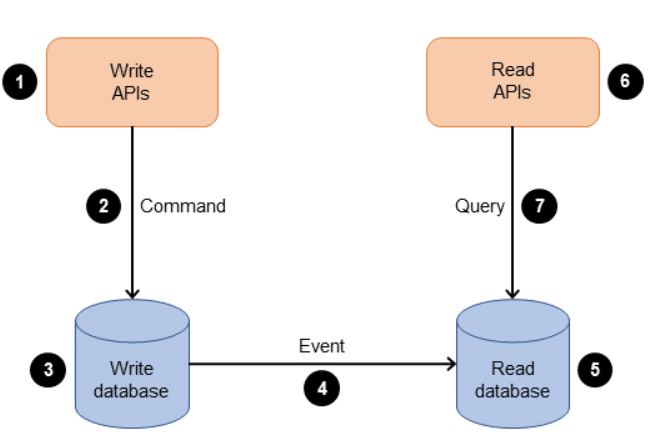
To sum up, these essential microservice design patterns help create robust, scalable, and maintainable systems by addressing various challenges like service discovery, fault tolerance, and independent scalability. Implementing these patterns effectively can lead to more resilient and efficient microservice architectures, enhancing overall system performance and reliability.