Introduction about Spring MVC (Model-View-Controller)
Spring MVC (Model-View-Controller) is a powerful framework for building Java-based web applications. Developed as part of the broader Spring Framework, Spring MVC provides a structured and modular approach to building scalable and maintainable web applications. In this article, we will delve into the key components, concepts, and best practices of Spring MVC.
Understanding the MVC Architecture:
Spring MVC follows the Model-View-Controller architecture, separating the application into three main components:
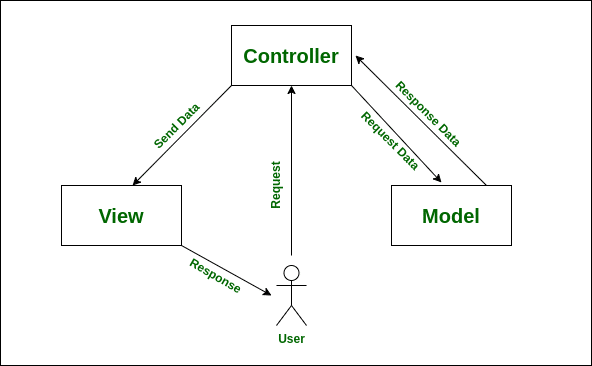
Model: Represents the application’s data and business logic.
View: Handles the presentation layer and user interface.
Controller: Manages the flow of data between the Model and View, handling user input and orchestrating the application’s behaviour.
Key Components of Spring MVC:
DispatcherServlet: Acts as the front controller, responsible for managing the entire request/response lifecycle. It dispatches requests to the appropriate controllers and views.
Controller: Handles user input, processes requests, and updates the Model. Controllers are annotated with @Controller and can utilize various annotations like @RequestMapping to map URLs.
Model: Represents the application’s data and business logic. The Model is typically populated by the Controller and sent to the View for presentation to the User.
ViewResolver: Resolves logical view names to actual views. Spring supports various view technologies, including JSP, Thymeleaf, and FreeMarker.
Request Handling in Spring MVC:
RequestMapping: Controllers use the @RequestMapping annotation to specify the URL patterns they can handle. It can be applied at both class and method levels.
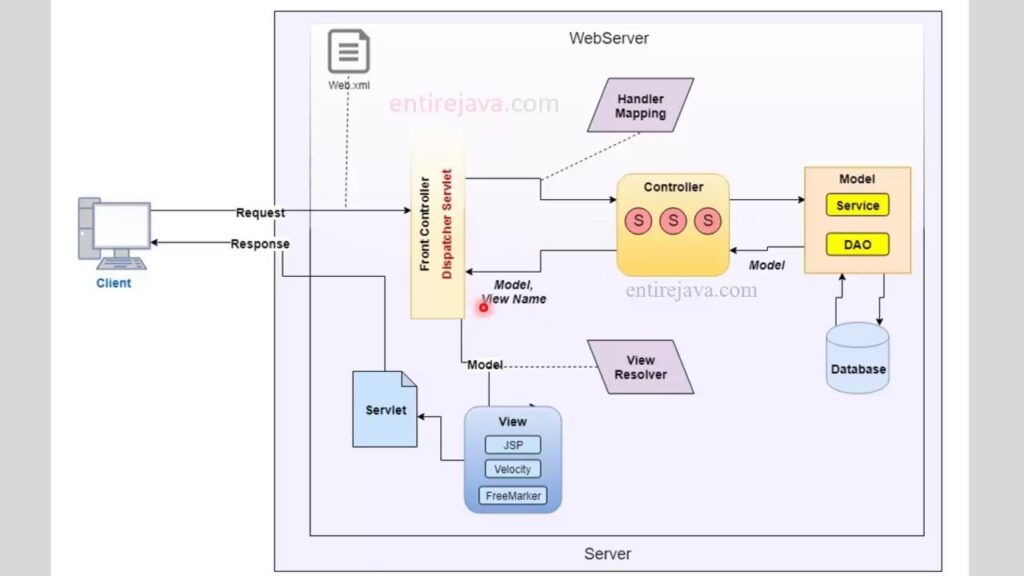
HTTP Methods: Controllers can be mapped to specific HTTP methods using annotations like @GetMapping, @PostMapping, etc.
Model and View Interaction:
Model Attributes: Data is transferred from the Controller to the View using model attributes. These attributes are added to the Model and can be accessed in the View.
View Rendering: Views are responsible for rendering the data to the client. Spring supports various view technologies, and developers can choose the one that best fits their requirements.
Form Handling:
@ModelAttribute: Used to bind form data to a method parameter or a method-level attribute.
Data Binding: Spring MVC supports automatic data binding between HTML form elements and Java objects.
Validation and Error Handling:
Validation Annotations: Spring MVC provides annotations like @Valid, to enable validation checking, and field validation annotations (e.g., @NotBlank, @Min, @Max) for input validation.
Error Handling: Spring MVC provides mechanisms to handle errors gracefully, including the use of @ExceptionHandler for handling specific exceptions.
Interceptors and Filters:
HandlerInterceptor: Interceptors allow pre-processing and post-processing of web requests. They can be used for tasks such as authentication, logging, or modifying the request/response.
Filter: Filters are applied to requests and responses before they reach the DispatcherServlet, allowing for more common request/response processing.
RESTful Web Services with Spring MVC:
Spring MVC can be used to build RESTful web services by leveraging annotations like @RestController and @RequestMapping.
Support for content negotiation, response status codes, and request parameters simplifies the development of RESTful APIs.
Testing Spring MVC Applications:
Spring MVC provides testing support through the MockMvc framework, enabling developers to write unit and integration tests for controllers.
Mock objects and test annotations facilitate the creation of comprehensive test suites.
Integration with Other Spring Projects:
Spring MVC seamlessly integrates with other Spring projects, such as Spring Security for authentication and authorization and Spring Data for database access.
The Spring Boot framework further simplifies the development of Spring MVC applications by providing conventions and defaults for configuration.
Introduction to Spring Boot
Spring Boot, an innovative project within the broader Spring Framework ecosystem, has revolutionized the way Java developers build and deploy applications. Born out of the need for a convention-over-configuration and opinionated framework, Spring Boot simplifies the complexities of traditional Spring development. In this article, we’ll explore the key features, benefits, and best practices of Spring Boot, shedding light on why it has become a go-to choice for building modern Java applications.
Introduction to Spring Boot:
Convention Over Configuration: Spring Boot embraces the convention-over-configuration paradigm, reducing the need for explicit configuration. It provides sensible defaults and eliminates boilerplate code, allowing developers to focus on business logic.
Opinionated Defaults: Spring Boot comes with opinionated defaults for libraries, frameworks, and configuration, minimizing the need for manual setup. This results in faster development cycles and reduces the burden of decision-making.
Creating Your First Spring Boot Application:
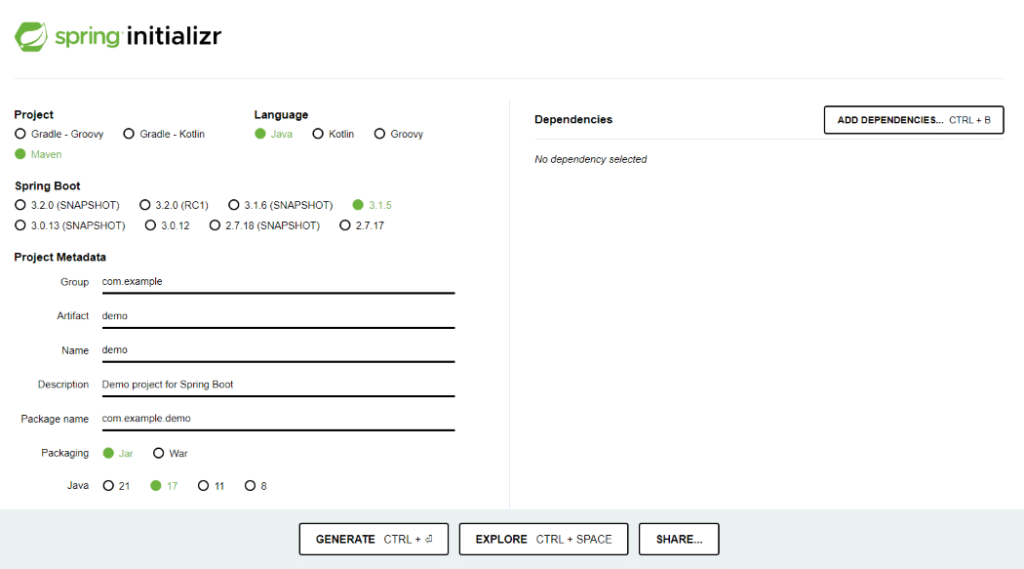
Spring Initializer: Developers can kickstart a Spring Boot project using the Spring Initializer, a web-based tool or integrated into popular IDEs, enabling the selection of dependencies, project settings, and packaging options.
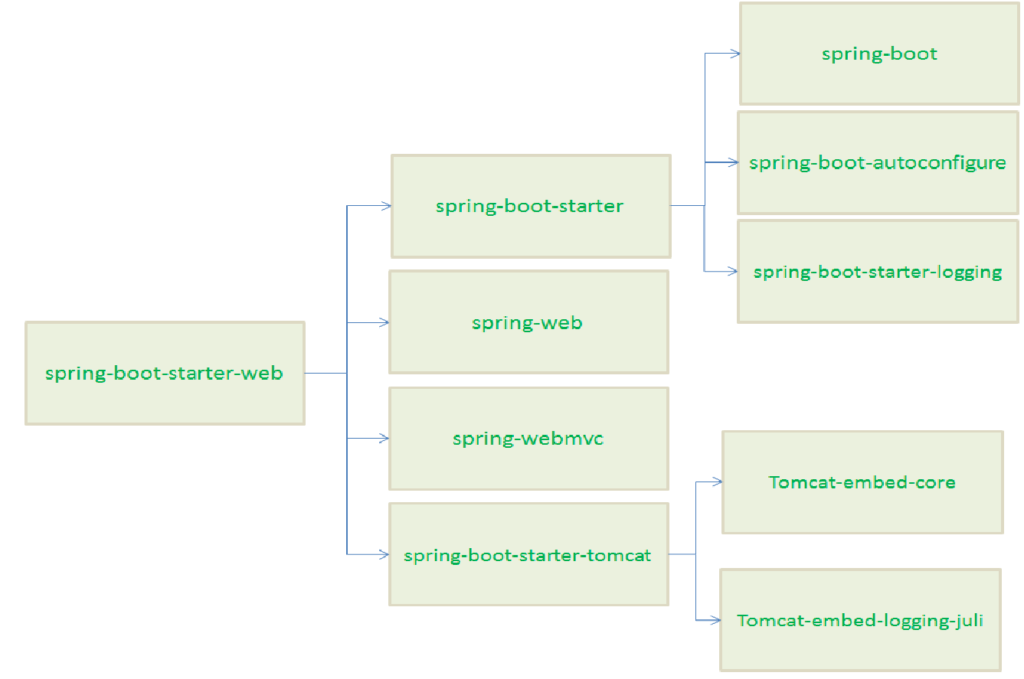
Starters: Spring Boot starters simplify dependency management by grouping common dependencies for specific tasks. For example, the spring-boot-starter-web includes everything needed to build a web application.
Auto-Configuration and Spring Boot Magic:
Auto-Configuration: Spring Boot’s auto-configuration feature eliminates the need for manual configuration by intelligently configuring the application based on the dependencies present in the classpath.
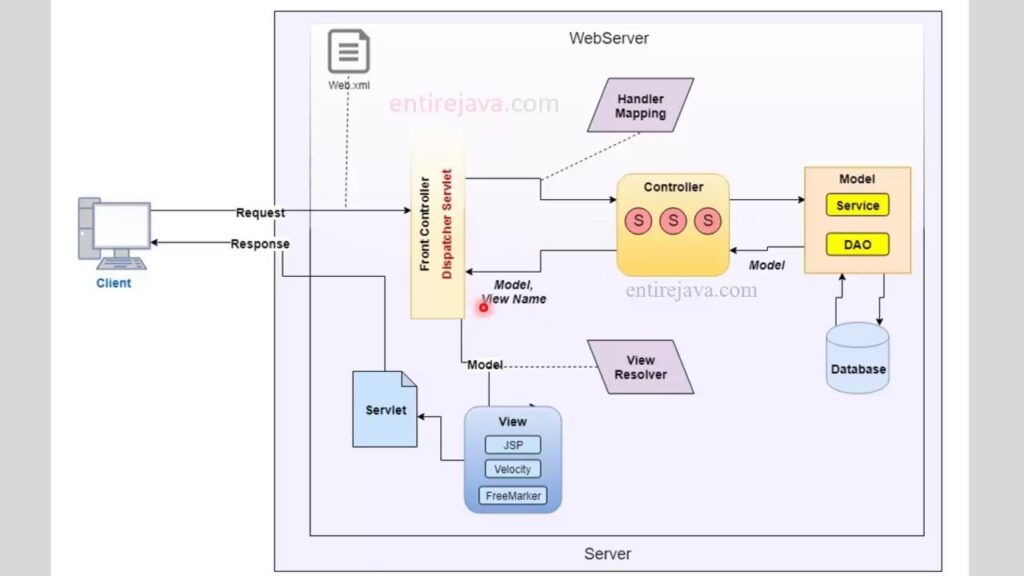
Spring Boot Magic: Developers often refer to the seamless setup provided by Spring Boot as “magic.” The framework anticipates requirements, reduces boilerplate, and streamlines development.
Embedded Web Servers and Microservices:
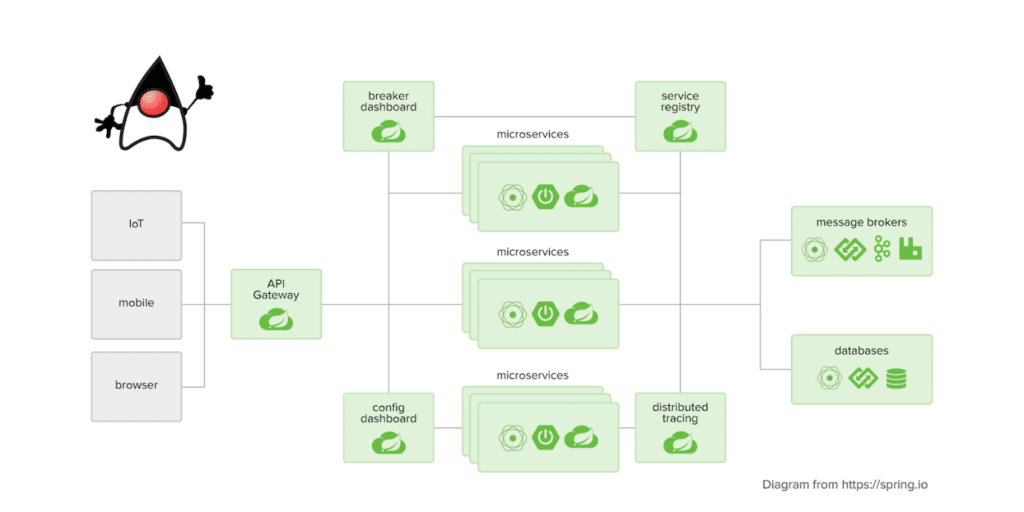
Embedded Servers: Spring Boot includes embedded web servers (Tomcat, Jetty, or Undertow) by default, simplifying deployment and reducing the need for external server setups.
Microservices Architecture: Spring Boot facilitates the development of microservices, allowing developers to create standalone, independently deployable services that communicate via lightweight protocols like HTTP or messaging.
Configuration Properties and Profiles:
Externalized Configuration: Spring Boot promotes externalized configuration, allowing developers to configure applications through properties files, YAML files, environment variables, or command-line arguments and can even be expanded to use a property server.
Profiles: Developers can use profiles to define different sets of configuration properties for different environments (e.g., development, testing, production).
Spring Boot Actuator: Monitoring and Management:
Actuator Endpoints: Spring Boot Actuator provides a set of production-ready features, including health, metrics, and info endpoints, allowing developers to monitor and manage applications in production.
Custom Endpoints: Developers can create custom actuator endpoints to expose application-specific information or management operations.
Spring Boot and Data Access:
Spring Data Integration: Spring Boot seamlessly integrates with Spring Data, simplifying data access through repositories and eliminating boilerplate code.
Database Auto-Configuration: Spring Boot’s auto-configuration extends to databases, making it easy to connect to different databases with minimal setup.
Testing in Spring Boot:
Test Support: Spring Boot provides comprehensive testing support, allowing developers to write unit tests, integration tests, and even full-blown integration tests using embedded servers.
@SpringBootTest: Annotating tests with @SpringBootTest initializes the Spring context, providing a realistic environment for testing.
Extending Spring Boot Applications:
Customization: While Spring Boot offers conventions and defaults, developers can easily customize and extend their applications by overriding configurations or adding additional components. Some of the example of customization annotations are @EnableScheduling, @EnableJpaRepositories, @EnableCaching.
Spring Boot Starters: Developers can create and share custom starters to encapsulate common configurations and dependencies within their organizations.
Spring Boot Ecosystem and Future Trends:
Spring Cloud: For building and deploying microservices at scale, Spring Boot integrates seamlessly with the Spring Cloud ecosystem, providing tools for service discovery, configuration management, and more.
Adoption and Community: Spring Boot’s popularity continues to grow, with a vibrant community and strong industry support. Regular updates and improvements ensure that developers stay on the cutting edge of Java application development.
Use Case:
Spring MVC: Ideal for developers who require fine-grained control over configurations and want to choose specific components for their web applications.
Spring Boot: Well-suited for developers who prefer convention-over-configuration, rapid development, and want to focus on business logic rather than extensive setup.
In summary:
While Spring MVC is a powerful and flexible web module within the Spring Framework, Spring Boot is a higher-level project that simplifies the development process, including web development using Spring MVC, by providing opinionated defaults and reducing boilerplate code. Developers often choose between Spring MVC and Spring Boot based on their project requirements, preferences, and the level of control they desire over the configuration and setup.