Introduction to Spring AI
Why do we need AI integration in Java applications?
We need AI integration in Java applications to make them smarter and more efficient. AI can automate tasks, process large amounts of data, and enhance user experiences by enabling features like chatbots, text analysis, image generation, voice recognition, and intelligent search.
What is Spring AI
Spring AI is an application framework for AI engineering that simplifies integration by seamlessly connecting enterprise data and APIs with AI models.
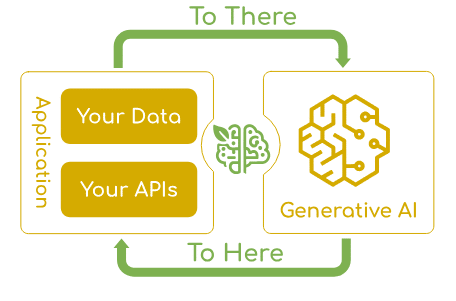
Key benefits and use cases.
🔹 Key Benefits
✅ Supports Multiple AI Providers – Works with OpenAI, Microsoft, Google, AWS, and more.
✅ AI Model Integration – Enables Chat, Text-to-Image, Embeddings, Audio Transcription, and Text-to-Speech.
✅ Vector Database Support – Compatible with Pinecone, Milvus, PostgreSQL, Redis, and many others.
✅ Easy API for AI & Vector Stores – Provides a unified API across AI models and databases.
✅ Function Calling & Real-time Actions – AI can execute custom functions as needed.
✅ Observability & AI Monitoring – Gain insights into AI operations and performance.
✅ Document Ingestion & Data Engineering – Helps process and transform data for AI models.
✅ AI Model Evaluation – Detects and prevents hallucinated responses.
✅ Spring Boot Auto Configuration – Seamless integration with Spring Boot apps.
✅ ChatClient API – Fluent API like WebClient for AI interactions.
✅ Advisors API – Optimizes and standardizes AI model interactions.
✅ Chat Memory & Retrieval-Augmented Generation (RAG) – Enhances AI responses with stored context.
🔹 Use Cases
AI-Powered Chatbots & Virtual Assistants 🤖
Smart Search & Recommendations 🔍
Automated Text Processing 📝
Image & Video AI Features 🎨🎥
Voice & Speech Applications 🎙️
Fraud Detection & Risk Analysis 🔐
AI-Powered Code Assistants & Dev Tools 🧑💻
Healthcare & Medical AI 🏥
Legal & Compliance Automation ⚖️
AI-Enhanced Learning & Education 📚
Key Concepts
✅ Prompts & Responses 📝 – AI interacts using user prompts (inputs) and model responses (outputs). Spring AI simplifies managing these conversations.
✅ OpenAiClient 🤖 – Connects to OpenAI’s API for text, image, and AI-powered features.
✅ ChatClient 💬 – Provides a unified interface for interacting with AI chat models, supporting text-based conversations.
✅ ImageClient 🖼️ – Enables AI-powered image generation and manipulation in applications.
✅ SpeechClient 🎙️ – Supports text-to-speech (TTS) and speech analysis for voice-based AI features.
✅ OutputParsers 🔄 – Converts AI responses into structured formats like Java objects, maps, and lists. (e.g., BeanOutputParser, MapOutputParser).
✅ PromptTemplate 📌 – Helps create dynamic prompts with placeholders, ensuring structured AI interactions.
✅ Embeddings 🔍 – Converts text into numerical vectors for tasks like semantic search, classification, and recommendations.
Setting Up Spring AI in a Spring Boot Project
To get started, let’s walk through the steps to integrate OpenAI into a Spring Boot project using Spring AI and your OpenAI API key [How to generate OpenAPI Key].
Add Dependencies
Add Dependencies to pom.xml configuration for different AI providers (OpenAI, Hugging Face, etc.). (spring-ai-openai, spring-ai-huggingface, etc.).
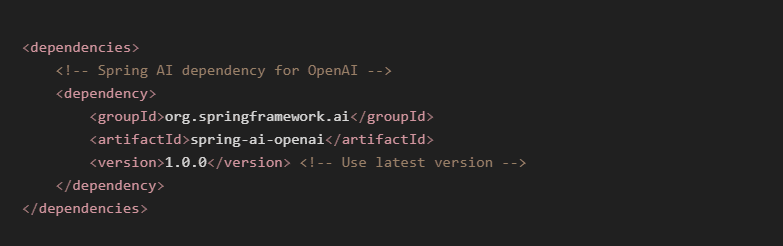
Set Up API Key (using application.yml or environment variables).

Add the following snippet to your SpringAiDemoApplication class:
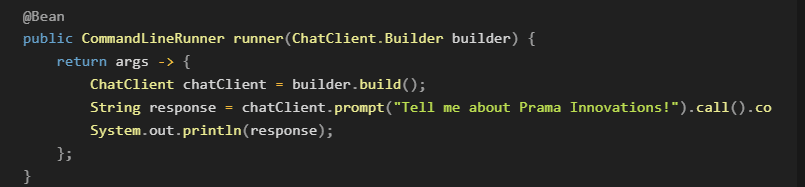
Run the application:
./mvnw spring-boot:run
Implementing Image & Audio AI Features 🎨🔊
Generating images using OpenAI’s DALL·E.
Create a REST controller that receives a text prompt, calls OpenAI’s API, and returns the generated image URL.
@RestController
class MyController {
@Autowired
private ImageModel imageModel;
@GetMapping(“/image/{prompt}”)
public Image getImage(@PathVariable String prompt){
ImageResponse response = imageModel.call(
new ImagePrompt(prompt,
OpenAiImageOptions.builder()
.withQuality(“hd”)
.withN(4)
.withHeight(1024)
.withWidth(1024).build())
);
return response.getResult().getOutput();
}
}
Sending Request to OpenAI’s DALL·E Model
- new ImagePrompt(prompt, options) – Creates an image generation request
- Quality: “hd” (high-definition image).
- Number of Images: 4 (generates four variations).
- Image Dimensions: 1024×1024 pixels.
- imageModel.call() – Calls OpenAI’s image generation API and retrieves the response.
- response.getResult().getOutput(); – Extracts and returns the generated image.
Speech-to-text conversion using Whisper AI
OpenAI’s Whisper model enables multilingual speech recognition and transcribes audio into JSON or text files.
Configuration in application.yaml
spring:
ai:
openai:
audio:
transcription:
options:
model: whisper-1
response-format: json # Options: json, text, srt, verbose_json, or vtt
temperature: 0 # Sampling temperature (0 to 1)
timestamp-granularities: segment # Options: segment, word (either or both)
prompt: “{prompt}” # Optional text to guide the model’s style or continue a previous audio segment.
language: “{language}” # Input audio language in ISO-639-1 format
Here’s a brief explanation of each parameter:
- model: Specifies the AI model to use for transcription. Here, whisper-1 is used for converting speech to text.
- response-format: Defines the output format of the transcription. Options include json, text, srt, verbose_json, or vtt.
- temperature: Controls the randomness in transcription output. A value of 0 ensures consistent results, while higher values (up to 1) introduce more variation.
- timestamp-granularities: Determines the level of timestamps in the output. Options include segment (for entire sections) or word (for individual words).
- prompt: An optional text prompt to guide the transcription style or provide context for continuing an audio segment.
- language: Specifies the expected language of the input audio in ISO-639-1 format (e.g., “en” for English, “es” for Spanish)
Supported Output Formats
- JSON – Structured format.
- Text – Plain text.
- SRT – Subtitle file.
- Verbose JSON – JSON with extra details.
- VTT – Subtitles for web videos.
Create rest controller that receives an audio file, sends it to OpenAI’s Whisper API for transcription, and returns the transcribed text. The transcription model is configured with language, prompt, and response format options
@RestController
@RequestMapping(“/ai”)
public class TranscriptionController {
private final OpenAiAudioTranscriptionModel transcriptionModel;
public TranscriptionController() {
OpenAiAudioTranscriptionOptions options = OpenAiAudioTranscriptionOptions.builder()
.withLanguage(“en”)
.withPrompt(“Create transcription for this audio file.”)
.withTemperature(0f)
.withResponseFormat(TranscriptResponseFormat.TEXT)
.build();
var openAiAudioApi = new OpenAiAudioApi(System.getenv(“OPENAI_API_KEY”));
this.transcriptionModel = new OpenAiAudioTranscriptionModel(openAiAudioApi, options);
}
@GetMapping(“/transcription”)
public String transcribeAudio(@Value(“classpath:speech.mp3”) Resource audioFile) {
AudioTranscriptionResponse response = transcriptionModel.call(new AudioTranscriptionPrompt(audioFile));
return response.getResult().getOutput();
}
}
Controller allows users to transcribe an audio file into text using OpenAI’s Whisper model via Spring AI. Here’s how it works:
Initializing OpenAI Transcription Model
- The constructor initializes the OpenAiAudioTranscriptionModel using OpenAI’s API key and predefined transcription options.
- OpenAiAudioTranscriptionOptions.builder() – Configures the transcription process:
- Language: “en” (English)
- Prompt: Provides context for the transcription.
- Temperature: 0f ensures deterministic (less random) results.
- Response Format: Text output.
- System.getenv(“OPENAI_API_KEY”) – Fetches the OpenAI API key from environment variables for secure access.
Handling Transcription Requests
- @Value(“classpath:speech.mp3”) Resource audioFile – Loads an audio file (speech.mp3) from the application’s classpath.
- transcriptionModel.call(new AudioTranscriptionPrompt(audioFile)) – Sends the audio file to OpenAI’s Whisper model for transcription.
- Returns the transcribed text as a response.
Real-World Use Cases & Future of Spring AI
Spring AI is transforming how developers integrate Artificial Intelligence (AI) into Java applications. Below are some of the key real-world use cases and the exciting future of Spring AI:
1. AI-powered Search Engines in Java Applications 🔍🤖
Use Case:
AI-powered search engines can enhance user experience by delivering more relevant and accurate search results. By integrating AI with search algorithms, Spring AI enables Java applications to understand user intent, context, and preferences.
- Example: A shopping website can use AI to suggest products based on past browsing patterns, ratings, and similar users’ preferences, making the search process more intuitive and personalized.
2. Automating Customer Support with AI Bots 🤖💬
Use Case:
AI bots, powered by Spring AI, can automate customer service tasks such as answering frequently asked questions, providing product information, or assisting with issue resolution in real-time. This significantly reduces response time and enhances customer satisfaction.
- Example: A telecom company can use an AI-powered chatbot to handle customer queries, troubleshoot issues, and even process service requests without requiring human agents to intervene.
3. AI-driven Recommendation Systems using Spring AI 🎯🛍️
Use Case:
Recommendation systems are commonly used in e-commerce, entertainment, and social media to suggest products, movies, music, or content based on user preferences. With Spring AI, Java applications can easily implement recommendation systems powered by AI models that analyze user behavior and deliver personalized recommendations.
- Example: A streaming service can use Spring AI to recommend shows or movies based on a user’s watch history and preferences, thereby improving engagement and content discovery.
The Future of Spring AI
As AI becomes more popular, Spring AI is making it easier for Java developers to use advanced AI technologies. With ongoing updates and support for more AI platforms, Spring AI is changing how intelligent apps are created and run.
Whether you’re new to AI or an experienced Java developer, Spring AI provides the tools you need to bring AI to your projects.
Conclusion: Harness the Power of AI with Spring AI
With Spring AI (1.0.0-SNAPSHOT), integrating advanced AI features into Java applications has never been easier. From chatbots to recommendation systems, the possibilities are endless!
Ready to start? Check out the GitHub repository for code samples and demos. Let’s build smarter apps together! 🚀
For more information and resources, check out the following links:
Feel free to reach out with your thoughts or questions. Happy coding! 👨💻👩💻